Building a Web Browser App for Blackberry Playbook Simulator
Updated on November 17, 2023
By Pete Freitag
By Pete Freitag

The blackberry playbook simulator doesn't yet include the web browser application, but I found it pretty easy to write my own using the qnx.media.QNXStageWebView
class and calling the loadUrl
method. This class also has a loadString(code, mimeType)
and executeJavaScript
method that looks interesting.
Here's the code for a simple browser:
package { import flash.display.Sprite; import flash.events.MouseEvent; import flash.geom.Rectangle; import flash.text.TextField; import qnx.media.QNXStageWebView; import qnx.ui.buttons.LabelButton; import qnx.ui.text.TextInput; // The following metadata specifies the size and properties of the canvas that // this application should occupy on the BlackBerry PlayBook screen. [SWF(width="1024", height="600", backgroundColor="#cccccc", frameRate="30")] public class PlaybookTest extends Sprite { private var addressInput:TextInput = null; private var webView:QNXStageWebView = null; public function PlaybookTest() { addressInput = new TextInput(); addressInput.x = 10; addressInput.y = 10; addressInput.text = "https://foundeo.com/"; var goButton:LabelButton = new LabelButton(); goButton.label = "Go"; goButton.x = addressInput.width + 10; goButton.y = addressInput.y; goButton.addEventListener(MouseEvent.CLICK, go); var closeButton:LabelButton = new LabelButton(); closeButton.label = "Close"; closeButton.addEventListener(MouseEvent.CLICK, closeWindow); closeButton.x = (stage.stageWidth - closeButton.width) - 10; closeButton.y = 10; webView = new QNXStageWebView(); webView.stage= stage; webView.autoFit=true; webView.viewPort = new Rectangle(10,100,stage.stageWidth,stage.stageHeight-100); webView.enableCookies = true; webView.enableJavascript = true; webView.enableScrolling = true; webView.loadURL("https://foundeo.com"); addChild(addressInput); addChild(goButton); addChild(closeButton); stage.nativeWindow.visible = true; } private function closeWindow(event:MouseEvent):void{ stage.nativeWindow.close(); } private function go(event:MouseEvent):void { webView.loadURL(addressInput.text); } } }
Here's a screenshot of the app:
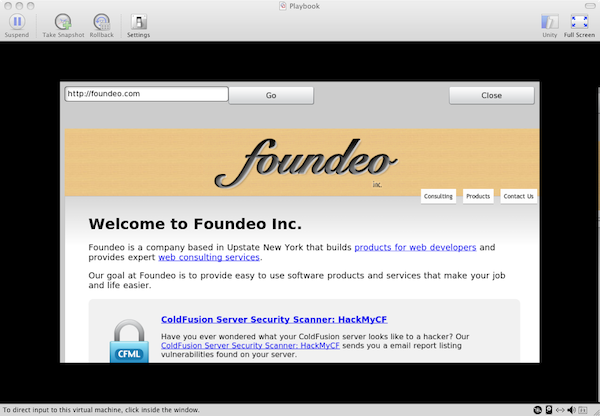
Building a Web Browser App for Blackberry Playbook Simulator was first published on November 17, 2010.
If you like reading about blackberry, playbook, mobile, air, or flex then you might also like:
- Getting Started with Blackberry Playbook App Development
- Blackberry WebWorks AJAX Problems
- Geolocation API for Adobe AIR?
Discuss / Follow me on Twitter ↯
Tweet Follow @pfreitagComments
I noticed that QNXStageWebView was not in the API docs (from what I can see). How did you find out the methods for it?
by Raymond Camden on 11/29/2010 at 3:17:29 PM UTC
Hi Ray, I found it by browsing through the "BlackBerry Tablet OS SDK 0.9.0 for Adobe AIR" library in the Package Explorer in Flash Buider.
I didn't realize it was undocumented :)
I didn't realize it was undocumented :)
by Pete Freitag on 11/29/2010 at 3:25:32 PM UTC
Nice - I keep forgetting we can do that. ;)
by Raymond Camden on 11/29/2010 at 3:35:54 PM UTC
You should be able to make a local URL request to
app://foo
where app:// represents the application install directory and "foo" is some file underneath it.
app://foo
where app:// represents the application install directory and "foo" is some file underneath it.
by Raymond Camden on 11/30/2010 at 12:00:04 PM UTC
Try app:/ notice it has ONE slash.
http://www.flex888.com/511/adobe-air-url-schemes-for-local-access.html
http://www.flex888.com/511/adobe-air-url-schemes-for-local-access.html
by Raymond Camden on 11/30/2010 at 12:37:35 PM UTC
Actually I may be wrong on that.
So - yesterday I was speaking with Brian Rinaldi. And he mentioned that he saw oddness using app:// syntax under Android. THat may be at play here.
Instead of using app://, you could use FIle.applicationDirectory.nativePath. That should give you a pointer to the directory - and then you just append the file name.
That's not _exactly_ the syntax, but you get the idea I hope.
So - yesterday I was speaking with Brian Rinaldi. And he mentioned that he saw oddness using app:// syntax under Android. THat may be at play here.
Instead of using app://, you could use FIle.applicationDirectory.nativePath. That should give you a pointer to the directory - and then you just append the file name.
That's not _exactly_ the syntax, but you get the idea I hope.
by Raymond Camden on 11/30/2010 at 12:51:00 PM UTC
Thanks for the useful discussion and comments Louis and Ray. I was interested in doing the same thing (to see if I could port a jQuery Mobile app), which is why I tried to figure out how to embed the browser in the first place. I also tried the app:// url without luck. I'll give the File.applicationDirectory.nativePath option a try when I get a chance.
by Pete Freitag on 11/30/2010 at 2:04:51 PM UTC
Hi Pete. I run into the same white page that Basti does. Did something change in the SDK that broke the Browser App code?
by CS on 01/14/2011 at 6:59:21 PM UTC
Hi Guys, yes this was with the first beta, so things must have changed since then. I'm not sure when I'll get a chance to try this again, but if anyone can find a workaround feel free to post it here.
by Pete Freitag on 01/19/2011 at 11:33:15 AM UTC